ESP32S31.14寸TFT QMI8658C
安装QMI8658C库
QMI8658C库安装,在库管理中搜索QMI8658,并安装SensorLib库.如下图所示.
Tips
QMI8658C 使用的IC2引脚 SDA 42 SCL 41
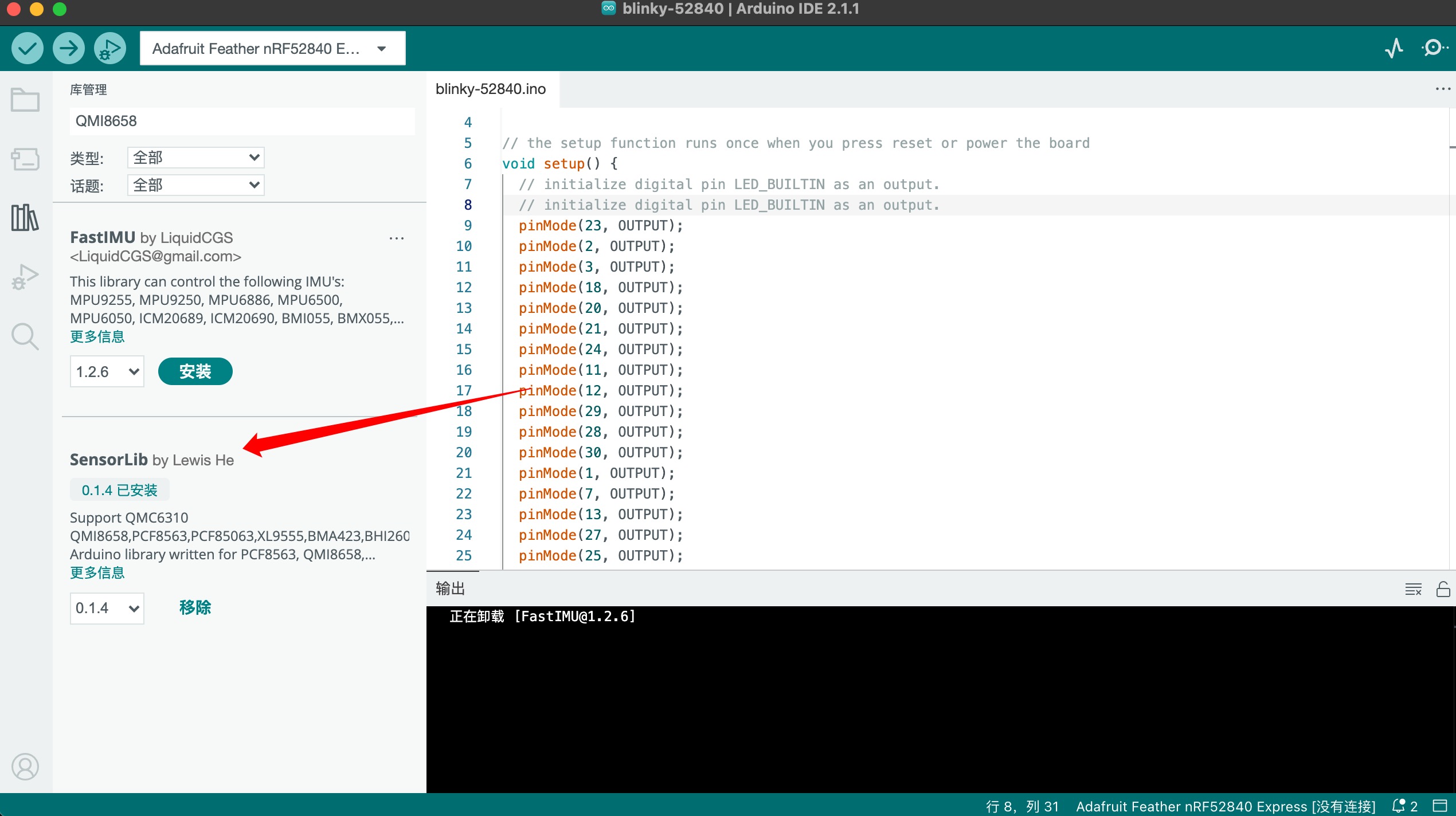
串口打印
示例代码
/**
*
* @license MIT License
*
* Copyright (c) 2022 lewis he
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*
* @file QMI8658_GetDataExample.ino
* @author Lewis He (lewishe@outlook.com)
* @date 2022-10-16
*
*/
#include <Arduino.h>
#include <Wire.h>
#include <SPI.h>
#include "SensorQMI8658.hpp"
#define USE_WIRE
#ifndef SENSOR_SDA
#define SENSOR_SDA 42
#endif
#ifndef SENSOR_SCL
#define SENSOR_SCL 41
#endif
#ifndef SENSOR_IRQ
#define SENSOR_IRQ -1
#endif
#define IMU_CS 5
SensorQMI8658 qmi;
IMUdata acc;
IMUdata gyr;
void setup()
{
Serial.begin(115200);
while (!Serial);
#ifdef USE_WIRE
//Using WIRE !!
if (!qmi.begin(Wire, QMI8658_L_SLAVE_ADDRESS, SENSOR_SDA, SENSOR_SCL)) {
Serial.println("Failed to find QMI8658 - check your wiring!");
while (1) {
delay(1000);
}
}
#else
if (!qmi.begin(IMU_CS)) {
Serial.println("Failed to find QMI8658 - check your wiring!");
while (1) {
delay(1000);
}
}
#endif
/* Get chip id*/
Serial.print("Device ID:");
Serial.println(qmi.getChipID(), HEX);
qmi.configAccelerometer(
/*
* ACC_RANGE_2G
* ACC_RANGE_4G
* ACC_RANGE_8G
* ACC_RANGE_16G
* */
SensorQMI8658::ACC_RANGE_4G,
/*
* ACC_ODR_1000H
* ACC_ODR_500Hz
* ACC_ODR_250Hz
* ACC_ODR_125Hz
* ACC_ODR_62_5Hz
* ACC_ODR_31_25Hz
* ACC_ODR_LOWPOWER_128Hz
* ACC_ODR_LOWPOWER_21Hz
* ACC_ODR_LOWPOWER_11Hz
* ACC_ODR_LOWPOWER_3H
* */
SensorQMI8658::ACC_ODR_1000Hz,
/*
* LPF_MODE_0 //2.66% of ODR
* LPF_MODE_1 //3.63% of ODR
* LPF_MODE_2 //5.39% of ODR
* LPF_MODE_3 //13.37% of ODR
* */
SensorQMI8658::LPF_MODE_0,
// selfTest enable
true);
qmi.configGyroscope(
/*
* GYR_RANGE_16DPS
* GYR_RANGE_32DPS
* GYR_RANGE_64DPS
* GYR_RANGE_128DPS
* GYR_RANGE_256DPS
* GYR_RANGE_512DPS
* GYR_RANGE_1024DPS
* */
SensorQMI8658::GYR_RANGE_64DPS,
/*
* GYR_ODR_7174_4Hz
* GYR_ODR_3587_2Hz
* GYR_ODR_1793_6Hz
* GYR_ODR_896_8Hz
* GYR_ODR_448_4Hz
* GYR_ODR_224_2Hz
* GYR_ODR_112_1Hz
* GYR_ODR_56_05Hz
* GYR_ODR_28_025H
* */
SensorQMI8658::GYR_ODR_896_8Hz,
/*
* LPF_MODE_0 //2.66% of ODR
* LPF_MODE_1 //3.63% of ODR
* LPF_MODE_2 //5.39% of ODR
* LPF_MODE_3 //13.37% of ODR
* */
SensorQMI8658::LPF_MODE_3,
// selfTest enable
true);
// In 6DOF mode (accelerometer and gyroscope are both enabled),
// the output data rate is derived from the nature frequency of gyroscope
qmi.enableGyroscope();
qmi.enableAccelerometer();
// Print register configuration information
qmi.dumpCtrlRegister();
Serial.println("Read data now...");
}
void loop()
{
if (qmi.getDataReady()) {
if (qmi.getAccelerometer(acc.x, acc.y, acc.z)) {
Serial.print("{ACCEL: ");
Serial.print(acc.x);
Serial.print(",");
Serial.print(acc.y);
Serial.print(",");
Serial.print(acc.z);
Serial.println("}");
}
if (qmi.getGyroscope(gyr.x, gyr.y, gyr.z)) {
Serial.print("{GYRO: ");
Serial.print(gyr.x);
Serial.print(",");
Serial.print(gyr.y );
Serial.print(",");
Serial.print(gyr.z);
Serial.println("}");
}
Serial.printf("\t\t\t\t > %lu %.2f *C\n", qmi.getTimestamp(), qmi.getTemperature_C());
}
delay(100);
}
代码讲解
qmi.getTimestamp()
qmi.getTimestamp(): 这个方法用于获取传感器的时间戳。时间戳通常是一个表示当前时间的数值,以毫秒或微秒为单位,具体取决于传感器的实现。时间戳在一些应用中可能用于计算时间间隔或同步数据。
qmi.getTemperature_C()
qmi.getTemperature_C(): 这个方法用于获取传感器的温度,返回的值是摄氏度(Celsius)。一些传感器提供温度信息,这对于一些应用来说是很有用的,例如在数据记录中考虑环境温度对传感器读数的影响。
qmi.getGyroscope(gyr.x, gyr.y, gyr.z)
qmi.getGyroscope(gyr.x, gyr.y, gyr.z) 是调用了 SensorQMI8658 类的方法,用于获取陀螺仪(gyroscope)的数据。让我们来解析这个调用.
SensorQMI8658 是一个类,可能包含了与 QMI8658 传感器通信和配置相关的方法。
getGyroscope 是一个方法,用于获取陀螺仪的数据。
(gyr.x, gyr.y, gyr.z) 是将获取到的陀螺仪数据存储到名为 gyr 的结构体或类中的属性 x、y 和 z 中。
一般情况下,这样的方法用于读取传感器的实时数据,例如陀螺仪返回的角速度信息。gyr.x、gyr.y 和 gyr.z 代表陀螺仪在三个轴上的角速度值。这些值的单位通常是角速度单位,比如度每秒(degrees per second)或弧度每秒(radians per second),具体取决于传感器的规格。
qmi.getAccelerometer(acc.x, acc.y, acc.z)
qmi.getAccelerometer(acc.x, acc.y, acc.z) 是调用了 SensorQMI8658 类的方法,用于获取加速度计(accelerometer)的数据。这个调用的解释与之前提到的获取陀螺仪数据的方法类似,让我们具体解析一下:
SensorQMI8658 是一个类,可能包含了与 QMI8658 传感器通信和配置相关的方法。
getAccelerometer 是一个方法,用于获取加速度计的数据。
(acc.x, acc.y, acc.z) 是将获取到的加速度计数据存储到名为 acc 的结构体或类中的属性 x、y 和 z 中。
一般情况下,这样的方法用于读取传感器的实时数据,例如加速度计返回的加速度信息。acc.x、acc.y 和 acc.z 代表加速度计在三个轴上的加速度值。这些值的单位通常是加速度单位,比如米每秒平方(meters per second squared)。
TFT显示
TFT显示需要先按照 之前的点亮TFT教程,安装TFT库,之后才能显示数据.
TFT 屏幕显示 加速度计、陀螺仪、时间戳、温度的数据
示例代码
/**
*
* @license MIT License
*
* Copyright (c) 2022 lewis he
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*
* @file QMI8658_GetDataExample.ino
* @author Lewis He (lewishe@outlook.com)
* @date 2022-10-16
*
*/
#include <Arduino.h>
#include <Wire.h>
#include <SPI.h>
#include "SensorQMI8658.hpp"
#include <TFT_eSPI.h>
TFT_eSPI tft;
#define USE_WIRE
#ifndef SENSOR_SDA
#define SENSOR_SDA 42
#endif
#ifndef SENSOR_SCL
#define SENSOR_SCL 41
#endif
#ifndef SENSOR_IRQ
#define SENSOR_IRQ -1
#endif
#define IMU_CS 5
SensorQMI8658 qmi;
IMUdata acc;
IMUdata gyr;
void setup()
{
Serial.begin(115200);
tft.begin();
// 设置屏幕旋转方向
tft.setRotation(1);
// 填充屏幕为黑色
tft.fillScreen(TFT_BLACK);
// 设置字体颜色
tft.setTextColor(TFT_WHITE);
// 设置文本大小为2倍
tft.setTextSize(2);
// 在屏幕上显示文本
tft.setCursor(20, 20);
#ifdef USE_WIRE
//Using WIRE !!
if (!qmi.begin(Wire, QMI8658_L_SLAVE_ADDRESS, SENSOR_SDA, SENSOR_SCL)) {
Serial.println("Failed to find QMI8658 - check your wiring!");
while (1) {
delay(1000);
}
}
#else
if (!qmi.begin(IMU_CS)) {
Serial.println("Failed to find QMI8658 - check your wiring!");
while (1) {
delay(1000);
}
}
#endif
/* Get chip id*/
Serial.print("Device ID:");
Serial.println(qmi.getChipID(), HEX);
qmi.configAccelerometer(
/*
* ACC_RANGE_2G
* ACC_RANGE_4G
* ACC_RANGE_8G
* ACC_RANGE_16G
* */
SensorQMI8658::ACC_RANGE_4G,
/*
* ACC_ODR_1000H
* ACC_ODR_500Hz
* ACC_ODR_250Hz
* ACC_ODR_125Hz
* ACC_ODR_62_5Hz
* ACC_ODR_31_25Hz
* ACC_ODR_LOWPOWER_128Hz
* ACC_ODR_LOWPOWER_21Hz
* ACC_ODR_LOWPOWER_11Hz
* ACC_ODR_LOWPOWER_3H
* */
SensorQMI8658::ACC_ODR_1000Hz,
/*
* LPF_MODE_0 //2.66% of ODR
* LPF_MODE_1 //3.63% of ODR
* LPF_MODE_2 //5.39% of ODR
* LPF_MODE_3 //13.37% of ODR
* */
SensorQMI8658::LPF_MODE_0,
// selfTest enable
true);
qmi.configGyroscope(
/*
* GYR_RANGE_16DPS
* GYR_RANGE_32DPS
* GYR_RANGE_64DPS
* GYR_RANGE_128DPS
* GYR_RANGE_256DPS
* GYR_RANGE_512DPS
* GYR_RANGE_1024DPS
* */
SensorQMI8658::GYR_RANGE_64DPS,
/*
* GYR_ODR_7174_4Hz
* GYR_ODR_3587_2Hz
* GYR_ODR_1793_6Hz
* GYR_ODR_896_8Hz
* GYR_ODR_448_4Hz
* GYR_ODR_224_2Hz
* GYR_ODR_112_1Hz
* GYR_ODR_56_05Hz
* GYR_ODR_28_025H
* */
SensorQMI8658::GYR_ODR_896_8Hz,
/*
* LPF_MODE_0 //2.66% of ODR
* LPF_MODE_1 //3.63% of ODR
* LPF_MODE_2 //5.39% of ODR
* LPF_MODE_3 //13.37% of ODR
* */
SensorQMI8658::LPF_MODE_3,
// selfTest enable
true);
// In 6DOF mode (accelerometer and gyroscope are both enabled),
// the output data rate is derived from the nature frequency of gyroscope
qmi.enableGyroscope();
qmi.enableAccelerometer();
// Print register configuration information
qmi.dumpCtrlRegister();
Serial.println("Read data now...");
}
void loop()
{
tft.fillScreen(TFT_BLACK);
tft.setCursor(0, 0);
if (qmi.getDataReady()) {
if (qmi.getAccelerometer(acc.x, acc.y, acc.z)) {
tft.print("{ACCEL: ");
tft.print(acc.x);
tft.print(",");
tft.print(acc.y);
tft.print(",");
tft.print(acc.z);
tft.print("} \n");
}
if (qmi.getGyroscope(gyr.x, gyr.y, gyr.z)) {
tft.print("{GYRO: ");
tft.print(gyr.x);
tft.print(",");
tft.print(gyr.y );
tft.print(",");
tft.print(gyr.z);
tft.print("} \n");
}
tft.printf(" > %lu %.2f *C\n", qmi.getTimestamp(), qmi.getTemperature_C());
}
delay(100);
}
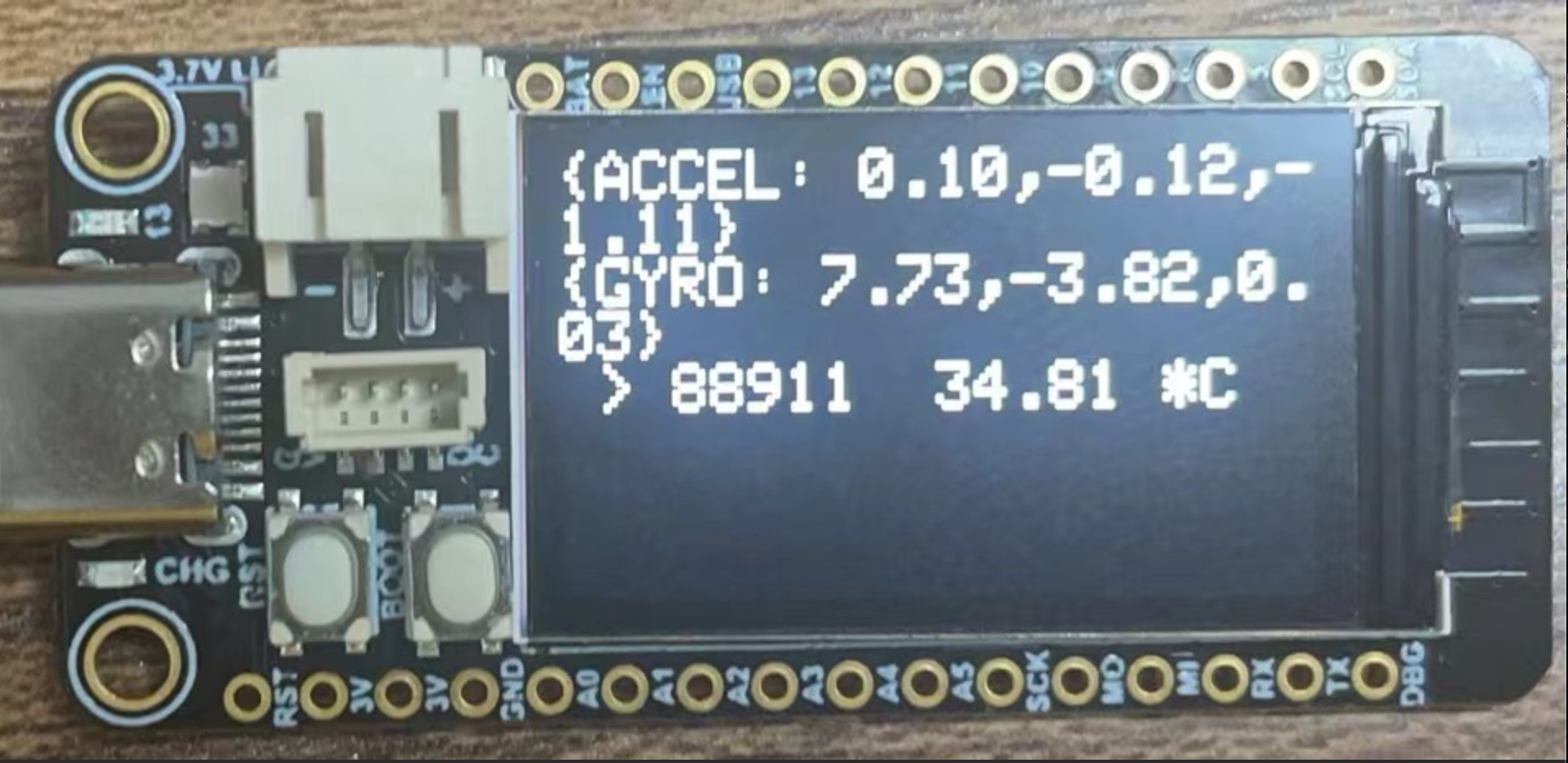